The Decorator pattern is a structural design pattern that enables you to dynamically attach new responsibilities or behaviors to objects without modifying their original structure. It is particularly useful when you need to extend the functionality of an existing class or interface without changing its existing code. In this article, we will delve into the Decorator pattern in .NET Core, its benefits, and how to implement it through a simple example.
The Decorator pattern comprises four main components:
Component: This is the interface or abstract class that defines the common behavior for all objects, encompassing both the primary object and the decorators.
Concrete Component: The primary object to which you want to add new behavior, implementing the Component interface or inheriting from the abstract class.
Decorator: This is an abstract class or interface that inherits from the Component and maintains a reference to the Component it wraps.
Concrete Decorator: The class that implements the Decorator, extending the functionality of the Concrete Component.
Benefits of the Decorator Pattern:
Flexibility: The Decorator pattern allows you to add or remove responsibilities from objects at runtime, making it easier to create different combinations of behaviors without modifying the original code.
Maintainability: By separating the concerns of the core object and its extended functionalities, the Decorator pattern promotes better code organization and maintainability.
Open/Closed Principle: The pattern adheres to the Open/Closed Principle, as it enables you to extend the functionality of a class without modifying its existing code.
Implementing the Decorator Pattern in .NET Core:
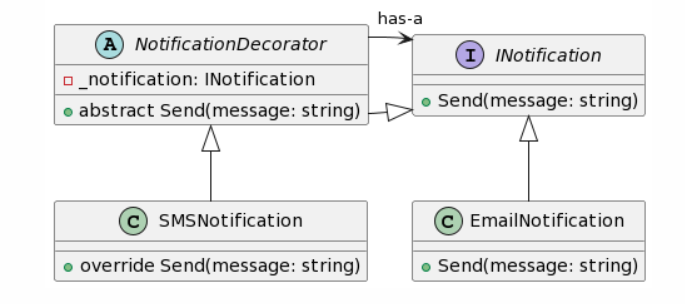
Let's implement a simple example of the Decorator pattern in .NET Core. In this scenario, we have a notification system that sends messages through various channels, such as email and SMS.
Define the Component interface:
public interface INotification
{
void Send(string message);
}
2. Create the Concrete Component:
public class EmailNotification : INotification
{
public void Send(string message)
{
Console.WriteLine($"Sending email: {message}");
}
}
3. Define the Decorator abstract class:
public abstract class NotificationDecorator : INotification
{
protected INotification _notification;
public NotificationDecorator(INotification notification)
{
_notification = notification;
}
public abstract void Send(string message);
}
4. Create the Concrete Decorator:
public class SMSNotification : NotificationDecorator
{
public SMSNotification(INotification notification) : base(notification) { }
public override void Send(string message)
{
_notification.Send(message);
Console.WriteLine($"Sending SMS: {message}");
}
}
5. Finally, use the Decorator pattern:
class Program
{
static void Main(string[] args)
{
INotification emailNotification = new EmailNotification();
INotification combinedNotification = new SMSNotification(emailNotification);
combinedNotification.Send("Hello, Decorator Pattern!");
}
}
In this example, the INotification interface represents the Component, EmailNotification is the Concrete Component, NotificationDecorator is the Decorator, and SMSNotification is the Concrete Decorator. The SMSNotification decorator can be used to extend the functionality of the EmailNotification object without modifying its original code.
The Decorator pattern is a powerful and flexible design pattern that allows you to extend the functionality of objects dynamically. By adhering to the Open/Closed Principle and promoting maintainability, the Decorator pattern is an excellent choice for situations where you need to add or modify behaviors without altering existing code. Implementing the Decorator pattern in .NET Core allows developers to leverage its benefits and create more adaptable and extensible software solutions.
Comentários